The Properties class represents a persistent set of properties. The Properties can be saved to a stream or loaded from a stream. Each key and its corresponding value in the property list is a string. - - - - Java™ SE 8 API
Java 里通过 Properties
类来读写 .properties
配置文件
.properties 文件
#
代表注释信息,其配置内容以键值对的形式出现,并且 String key, String value
1 | #Fri Jun 16 14:48:31 CST 2017 |
school 的值是中文,这里是 Unicode
编码的形式,在 IDEA 中想要以中文的形式浏览,需要进行以下操作:
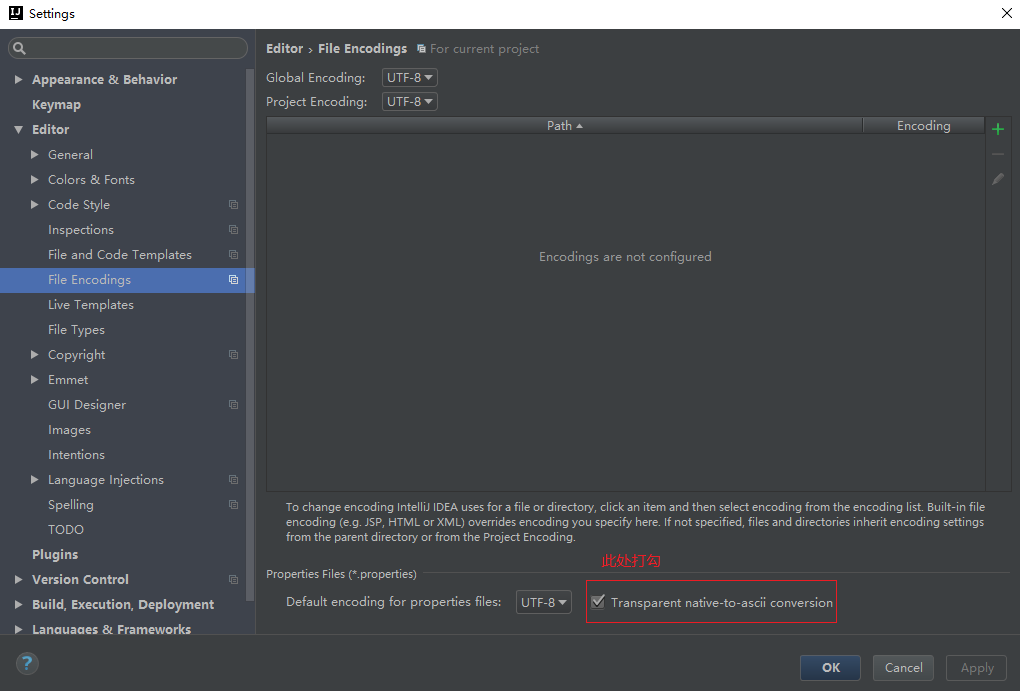
实例
1 | import java.io.*; |
源码分析
1 | public class Properties extends Hashtable<Object,Object> { |