The Trips table holds all taxi trips. Each trip has a unique Id, while Client_Id and Driver_Id are both foreign keys to the Users_Id at the Users table.
Status is an ENUM type of (‘completed’, ‘cancelled_by_driver’, ‘cancelled_by_client’).
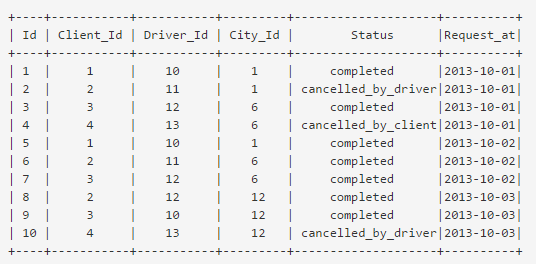
The Users table holds all users. Each user has an unique Users_Id, and Role is an ENUM type of (‘client’, ‘driver’, ‘partner’).
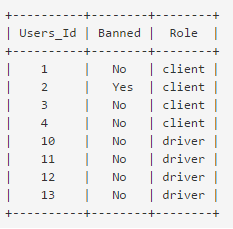
Write a SQL query to find the cancellation rate of requests made by unbanned clients between Oct 1, 2013 and Oct 3, 2013. For the above tables, your SQL query should return the following rows with the cancellation rate being rounded to two decimal places.
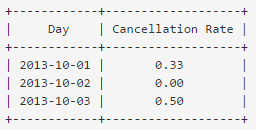
描述
在 unbanned clients
的前提下,计算出 Oct 1, 2013 and Oct 3, 2013
这段时间内,每一天,Status
不为 completed
的数目所占的比例
代码
1 | -- 方法一 |
1 | -- 方法二 |
1 | -- 方法三 |