The longest common subsequence (LCS) problem is the problem of finding the longest subsequence common to all sequences in a set of sequences (often just two sequences). It differs from problems of finding common substrings: unlike substrings, subsequences are not required to occupy consecutive positions within the original sequences. The longest common subsequence problem is a classic computer science problem, the basis of data comparison programs such as the diff utility, and has applications in bioinformatics. It is also widely used by revision control systems such as Git for reconciling multiple changes made to a revision-controlled collection of files. - - - - wikipedia
描述
最长公共子序列,所求得的字符串在原字符串中可以不连续
分析
动态规划思想,以一个二维数组来存储子串长度
由于可以不连续,所以在当前字符不匹配时,记录 Math.max(L[i - 1][j], L[i][j - 1])
,而不是清零
流程:
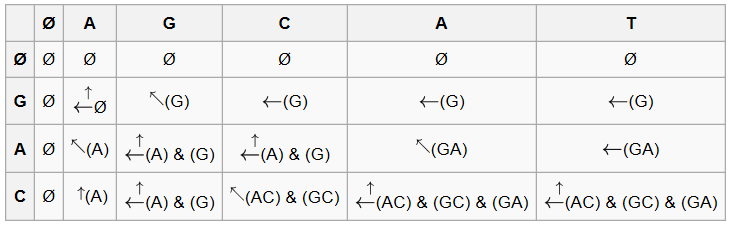
回溯:以长度来代替序列,生成二维数组之后,从 L[S.length() - 1][T.length() - 1]
开始回溯,输出 LCS(或 LCSs)
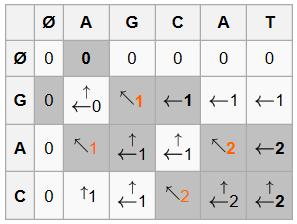
差异:通过回溯,还可以知道字符串之间的差异。
![[ -X,M,+Z,J,-Y,A,+W,+X,U,-Z ]](/images/longest-common-subsequence-problem-7.png)
代码
1 | import java.util.HashSet; |