Implement next permutation, which rearranges numbers into the lexicographically next greater permutation of numbers.
If such arrangement is not possible, it must rearrange it as the lowest possible order (ie, sorted in ascending order).
The replacement must be in-place, do not allocate extra memory.
Here are some examples. Inputs are in the left-hand column and its corresponding outputs are in the right-hand column.1,2,3 → 1,3,2
3,2,1 → 1,2,3
1,1,5 → 1,5,1
描述
给出当前序列的下一个序列(要求得到的序列,仅仅只比当前序列大),若当前序列为降序,则输出升序
分析
- 当序列是降序时(最大序列),不存在比这个序列大的序列
- 当序列是升序时(最小序列),不存在比这个序列小的序列
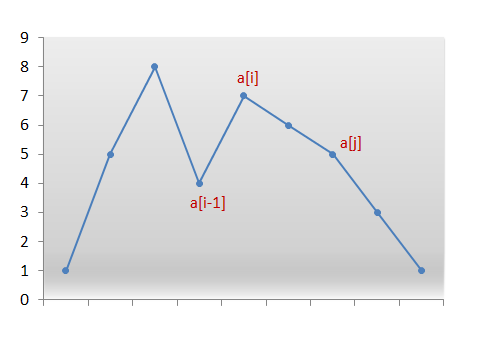
如图所示,假设序列长度为 len
,a[i] ~ a[len-1]
是降序序列,即最大的序列。当 a[i-1] < a[i]
时,先从序列 a[i-1] ~ a[len-1]
中选取一个数(仅仅只大于 a[i-1]
),与 a[i-1]
交换,那么此时序列已经比原序列要大,之后只需要将 a[i] ~ a[len-1]
序列变成升序序列,即使 a[i] ~ a[len-1]
为最小序列
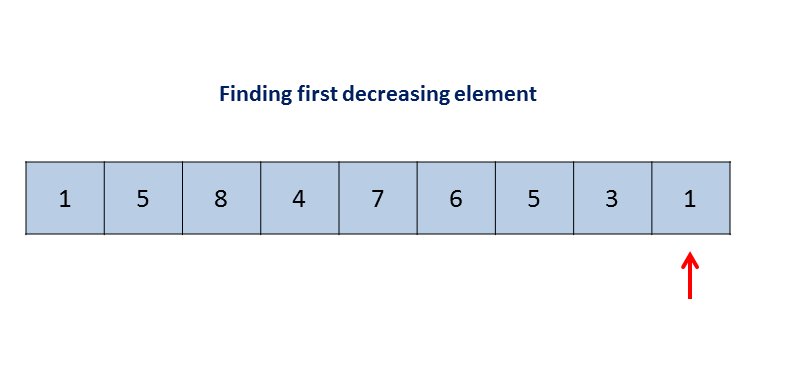
代码
1 | public class Solution { |