Given n non-negative integers representing an elevation map where the width of each bar is 1, compute how much water it is able to trap after raining.
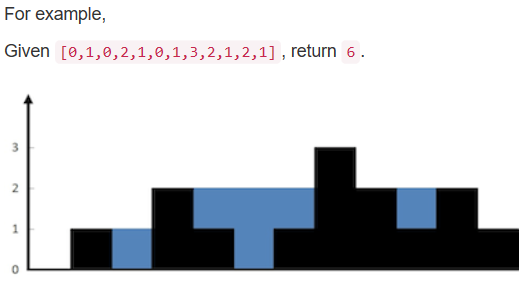
描述
给定 n
个非负整数,求图中蓝色部分
分析
每个值,其实都是由其左右的最高点 maxleft
,maxright
所决定的,即 min(maxleft , maxright) - height[i]
代码
1 | public class Solution { |