Given a binary tree and a sum, determine if the tree has a root-to-leaf path such that adding up all the values along the path equals the given sum.
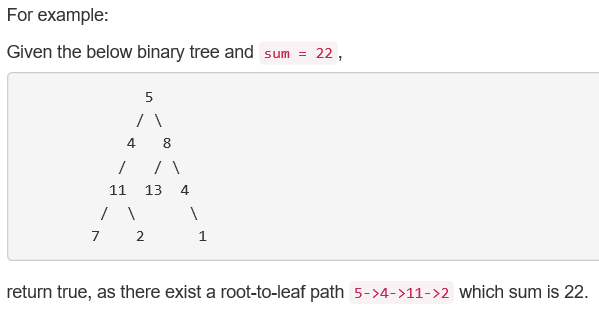
描述
判断是否有一条路径(从根节点到叶子节点)的和为 sum
分析
类似于树的前序遍历
代码
1 | /** |
Given a binary tree and a sum, determine if the tree has a root-to-leaf path such that adding up all the values along the path equals the given sum.
判断是否有一条路径(从根节点到叶子节点)的和为 sum
类似于树的前序遍历
1 | /** |