Populate each next pointer to point to its next right node. If there is no next right node, the next pointer should be set to NULL.Initially, all next pointers are set to NULL.
What if the given tree could be any binary tree?
Note:
You may only use constant extra space.
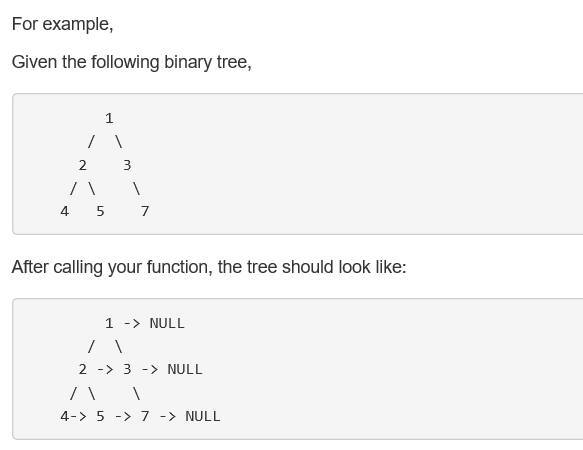
描述
给出一个任意的二叉树,使其每一个节点都指向右侧节点,并且不能使用额外的空间进行操作
分析
设置两个指针,分别指向相邻的两层,通过上层的节点,将下层的节点连接起来
代码
1 | /** |