The heapsort algorithm involves preparing the list by first turning it into a max heap. The algorithm then repeatedly swaps the first value of the list with the last value, decreasing the range of values considered in the heap operation by one, and sifting the new first value into its position in the heap. This repeats until the range of considered values is one value in length. - - - - wikipedia
The steps are:
- Call the buildMaxHeap() function on the list. Also referred to as heapify(), this builds a heap from a list in $O(n)$ operations.
- Swap the first element of the list with the final element. Decrease the considered range of the list by one.
- Call the siftDown() function on the list to sift the new first element to its appropriate index in the heap.
- Go to step (2) unless the considered range of the list is one element.
The buildMaxHeap() operation is run once, and is $O(n)$ in performance. The siftDown() function is $O(log(n))$, and is called $n$ times. Therefore, the performance of this algorithm is $O(n + n * log(n))$ which evaluates to $O(n log n)$.
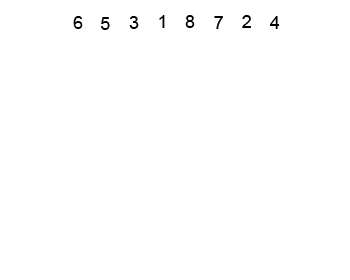
分析
首先将列表变为大根堆,之后将根节点和最后一个叶子节点进行交换,堆大小减一,再维护堆,重复操作,直至大根堆为空,此时列表已经有序
这里建堆采用 siftDown()
的方式,从后(最后一个非叶子节点)往前(根节点)遍历,这过程中,使得每一个子堆都满足要求,直至整个堆满足要求
特点
- 基于完全二叉树
- 不稳定的排序
- 时间复杂度(最佳): $O(n log(n))$
- 时间复杂度(平均): $O(n log(n))$
- 时间复杂度(最差): $O(n log(n))$
- 空间复杂度(最差): $O(1)$
auxiliary
代码
1 | import java.util.Scanner; |