Conceptually, a merge sort works as follows: - - - wikipedia
- Divide the unsorted list into n sublists, each containing 1 element (a list of 1 element is considered sorted).
- Repeatedly merge sublists to produce new sorted sublists until there is only 1 sublist remaining. This will be the sorted list.
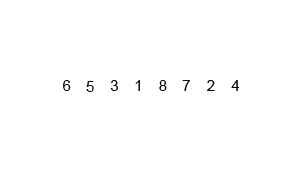
分析
采用自顶向下的方式,利用递归将列表分割成一个个子列表,直至所有的子列表的大小都为 1
,然后再将左右相邻的子列表合并,直至所有的子列表都合并成一个列表。代码中采用 temp
数组来充当临时数组,存储子列表合并后的结果,再将其覆盖到原列表上
特点
- 稳定的排序
- 时间复杂度(最佳): $O(n log(n))$
- 时间复杂度(平均): $O(n log(n))$
- 时间复杂度(最差): $O(n log(n))$
- 空间复杂度(最差): $O(n)$
auxiliary
代码
1 | import java.util.Arrays; |